Palindrome Program In Python
In this article, you will learn how to create a palindrome program in python using multiple different methods for both strings and numbers.
- Palindrome Introduction
- String Palindrome Program In Python
- Number Palindrome Program In Python
- Conclusion
Table Of Contents
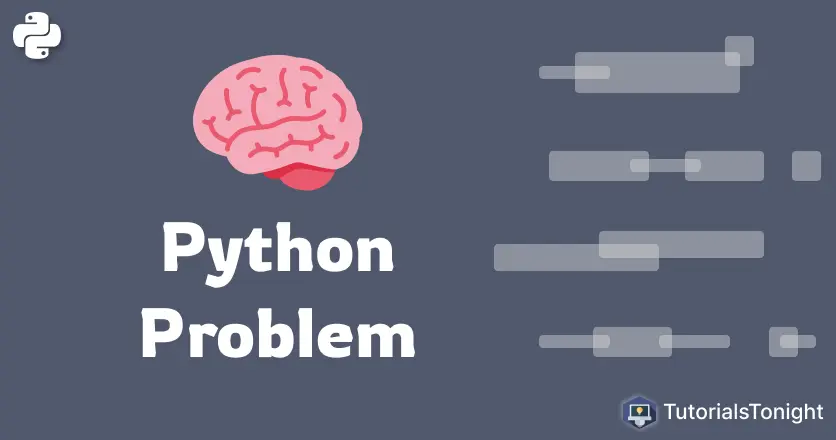
Palindrome Introduction
Any series of characters can be considered a palindrome if it is the same when reversed. For example, the following are palindromes:
MADAM ⟺ MADAM ROTATOR ⟺ ROTATOR REFER ⟺ REFER 1234321 ⟺ 1234321 11:11:11 ⟺ 11:11:11
The concept of a palindrome is used in finding a similar sequence in DNA and RNA.
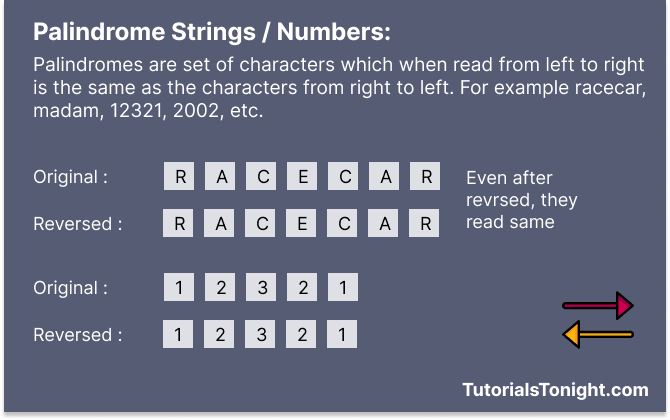
String Palindrome Program In Python
Now you understand palindromes. Let's create Python programs that tell whether a given string is a palindrome or not.
There can be many different methods to check if a string is a palindrome. We are going to discuss 3 different methods in this article.
Method 1: Find String Palindrome
You know that any string which is a palindrome must get the same character either from the left or right side. So we can use this concept and match characters from the left and right sides. If we get a match then we can move to the next character. If we don't get a match then the string is not a palindrome.
For this take 2 variables one starts at 0 and one at the start at the end of the string. We will keep on comparing characters from the left and right sides by moving towards the middle using a while loop in python.
Here is the Python program using this concept:
# take a string as input
str = input("Enter the string: ")
def isPalindrome(s):
i = 0
j = len(s) - 1
while i < j:
if s[i] != s[j]:
return False
i += 1
j -= 1
return True
if isPalindrome(str):
print(str + " is a palindrome")
else:
print(str + " is not a palindrome")
Output:
Enter the string: refer refer is a palindrome Enter the string: abcde abcde is not a palindrome
Code Explanation:
- Start the program by taking input from the user.
- Create a Python function that returns True if the string is a palindrome and False if the string is not a palindrome.
- The isPalindrome() (our function) executes a while loop and checks if characters from the left and right sides are equal. If they are equal then we move to the next character. If they are not equal then the string is not a palindrome.
- Use the python if-else statement to check and print results accordingly.
Method 2: Reverse String
Another method could be by reversing the string and then comparing it with the original string. If they are the same, then the string is a palindrome.
# take a string as input
str = input("Enter the string: ")
def isPalindrome(s):
return s == s[::-1]
if isPalindrome(str):
print(str + " is a palindrome")
else:
print(str + " is not a palindrome")
Output:
Enter the string: racecar racecar is a palindrome Enter the string: cricket cricket is not a palindrome
Code Explanation:
- This code is very simple. Python creates the reverse of string by s[::-1]. s[::-1] means taking the string from start to end and -1 means taking the string in reverse order.
- Now we have the reverse of the string and can compare it with the original string. If they are equal then the string is a palindrome.
Method 3: Recursive Method
Another method is to use recursion to check if the string is a palindrome or not. In each iteration, we can pass the string without the first character and last character. If we get a match then we can move to the next character. If we don't get a match then the string is not a palindrome.
# take a string as input
str = input("Enter the string: ")
def isPalindrome(s):
if len(s) <= 1:
return True
if s[0] != s[-1]:
return False
return isPalindrome(s[1:-1])
if isPalindrome(str):
print(str + " is a palindrome")
else:
print(str + " is not a palindrome")
Output:
Enter the string: racecar racecar is a palindrome Enter the string: Book Book is not a palindrome
Code Explanation:
- This program uses recursion to solve the problem. The function isPalindrome() checks the length of the string and if it is less than or equal to 1 then it returns True. If the first and last character is not equal then it returns False.
- After that, if non of the above condition is true then it calls itself again by passing string without the first and last character.
- Then the same process is repeated until we get a match or we reach the end of the string.
Number Palindrome Program In Python
We can't directly access individual digits of a number. So we need to use some logic to access individual digits of a number.
Look at these methods to find if a number is palindrome or not:
Method 1: Creating New Number In Reverse Order
In this method, we will create a new number by reversing the position of digits of the original number.
# take a string as input
num = int(input("Enter the number: "))
def isPalindrome(num):
if num < 0:
return False
else:
temp = num
rev = 0
while temp > 0:
rev = rev * 10 + temp % 10
temp = temp // 10
if num == rev:
return True
else:
return False
if isPalindrome(num):
print(str(num) + " is a palindrome")
else:
print(str(num) + " is not a palindrome")
Output:
Enter the number: 12321 12321 is a palindrome Enter the number: 12345 12345 is not a palindrome
Code Explanation:
- The function isPalindrome() checks if the number is less than 0 then it returns False. Because a negative number is not a palindrome.
- Now create a temporary variable and assign a number to it. Also, create a variable to store the reverse of the number.
- Now Execute a while loop and check if the temporary variable is greater than 0. If it is then we can continue.
- In each iteration, multiple reverse variables by 10 and add the remainder of the temporary variable to the reverse variable.
- After that, we divide the temporary variable by 10 to get the next digit.
- If the number and reverse variable are equal then it is a palindrome otherwise it is not a palindrome.
Method 2: Convert number to string
Another method is to convert the number to string and then use any of the above discussed methods to check if the number is a palindrome.
# take a string as input
num = int(input("Enter the number: "))
def isPalindrome(num):
s = str(num)
return s == s[::-1]
if isPalindrome(num):
print(str(num) + " is a palindrome")
else:
print(str(num) + " is not a palindrome")
Output:
Enter the number: 1234321 1234321 is a palindrome Enter the number: 1234 1234 is not a palindrome
Conclusion
In this brief guide, we have discussed how to check if a string or number is a palindrome or not using 5 different methods.
Above we have seen 5 palindrome program in Python using different approaches. Don't stop learning here check these out: Armstrong Number in Python, Factorial Program in Python, Fibonacci Sequence in Python.