List Comprehension in Python
In this tutorial, you are going to learn about list comprehension in Python in the easiest way possible with a lot of examples.
- List Comprehension
- Nested List Comprehension
- List Comprehension with if condition
- For Loop vs List Comprehension
- Examples
- Quiz
- Conclusion
Table Of Contents
List Comprehension
List comprehension is a very powerful feature of Python. It is used to create a new list from an existing list in Python. It is also used to create a list from other iterables like strings, tuples, arrays, etc.
It is generally more compact and faster than normal functions and loops for creating lists.
For example, to create a list of squares of numbers from 1 to 5, we can use a for loop as follows:
numbers = [1, 2, 3, 4, 5]
squares = []
for i in numbers:
squares.append(i**2)
print(squares)
# output: [1, 4, 9, 16, 25]
But we can also use list comprehension to do the same task in a single line as follows:
numbers = [1, 2, 3, 4, 5]
# List comprehension
squares = [i**2 for i in numbers]
print(squares)
# output: [1, 4, 9, 16, 25]
It consists of brackets containing an expression followed by a for clause, then zero or more for or if clauses. The expressions can be anything, meaning you can put all kinds of objects in lists.
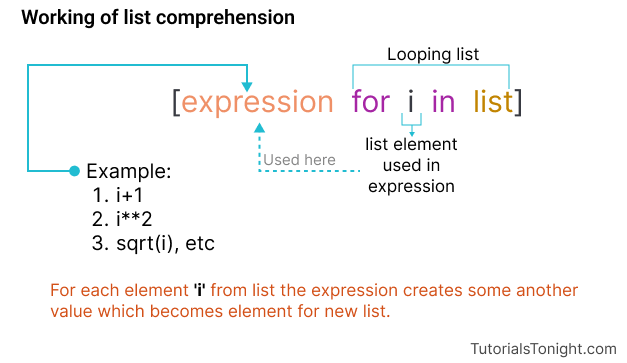
Syntax
Here is the simple syntax of list comprehension:
[expression for item in list (if condition)]
Here, expression
is the python expression that is executed for each item in the list. item
is the element in the list. list
is the list you want to iterate over.
Example
Let's see an example to understand it better.
Create a list with 2× each element in the list.
- List comprehension
- For loop
num = [1, 2, 3, 4, 5]
# List comprehension
doubled = [i*2 for i in num]
print(doubled)
# output: [2, 4, 6, 8, 10]
num = [1, 2, 3, 4, 5]
doubled = []
# For loop
for i in num:
doubled.append(i*2)
print(doubled)
# output: [2, 4, 6, 8, 10]
Create a list of characters from a given string.
- List comprehension
- For loop
str = "Hello World"
# List comprehension
chars = [i for i in str]
print(chars)
# output: ['H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd']
str = "Hello World"
chars = []
# For loop
for i in str:
chars.append(i)
print(chars)
# output: ['H', 'e', 'l', 'l', 'o', ' ', 'W', 'o', 'r', 'l', 'd']
Create a list with only even numbers from a given list.
- List comprehension
- For loop
num = [1, 2, 3, 4, 5]
# List comprehension
even = [i for i in num if i%2==0]
print(even)
# output: [2, 4]
num = [1, 2, 3, 4, 5]
even = []
# For loop
for i in num:
if i%2==0:
even.append(i)
print(even)
# output: [2, 4]
Nested List Comprehension
Just like nesting loops in Python, we can also nest list comprehensions in Python. It can be used to create a list of lists.
You can use nesting when you need to run a loop for each element in a list.
For example, let's say we want to create a list of all characters from a list of strings.
strList = ["Hello", "World"]
# Nested list comprehension
chars = [char for string in strList for char in string]
print(chars)
# output: ['H', 'e', 'l', 'l', 'o', 'W', 'o', 'r', 'l', 'd']
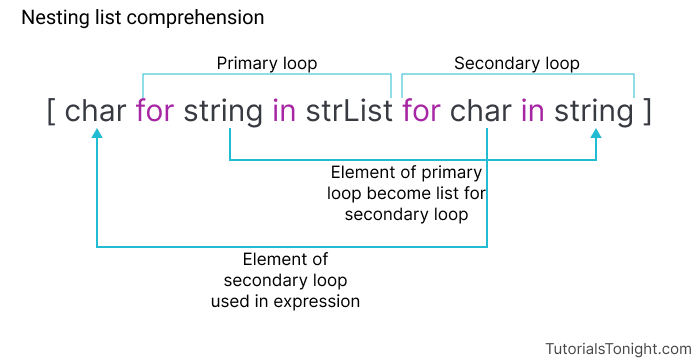
Let's see another example to understand it better.
Create a list of lists with elements starting from 1 to 3, and each list should have 3 elements.
- List comprehension
- For loop
# List comprehension
list = [[i for i in range(1, 4)] for j in range(3)]
print(list)
# output: [[1, 2, 3], [1, 2, 3], [1, 2, 3]]
list = []
# For loop
for j in range(3):
row = []
for i in range(1, 4):
row.append(i)
list.append(row)
print(list)
# output: [[1, 2, 3], [1, 2, 3], [1, 2, 3]]
List Comprehension with if condition
if statement executes a block of code if the condition is true. We can use if statement in list comprehension to create a list based on a condition.
For example, let's say we want to create a list of even numbers from a given list.
num = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# List comprehension with if condition
even = [i for i in num if i%2==0]
print(even)
# output: [2, 4, 6, 8, 10]
Here is another example of creating a list of strings starting with 'j' and ignoring the case.
strList = ["HTML", "C++", "Python", "JavaScript", "Java"]
# List comprehension with if condition
jStr = [i for i in strList if i.lower().startswith('j')]
print(jStr)
# output: ['JavaScript', 'Java']
if-else condition
You can also use else with if statement in the list comprehension.
num = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# List comprehension with if-else condition
newNum = [i+1 if i%2==0 else i+2 for i in num]
print(newNum)
# output: [3, 3, 5, 5, 7, 7, 9, 9, 11, 11]
For loop vs List Comprehension
Both for loop and list comprehension can be used to create a list. But, there are some differences between them.
For loop | List comprehension |
---|---|
It is used to iterate over a sequence. | It is used to create a list. |
It is more flexible than list comprehension. | It is more concise than for loop. |
It is more readable than list comprehension. | It is less readable than for loop. |
It is slower than list comprehension. | It is faster than for loop. |
It is used when you need to perform some operations on each element of a list, one of the operations is to create a list. Means loop covers way more than just creating a list. | It is used when you need to create a list based on a condition. Means list comprehension covers only creating a list. |
Note: All list comprehension can be written using for loop, but not all for loop can be written using list comprehension.
Examples
Let's see some examples of list comprehension.
Example 1:
Create a list of numbers from 1 to 10.
nums = [i for i in range(1, 11)]
print(nums)
# output: [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
Example 2:
Create a list of even numbers from 1 to 10.
nums = [i for i in range(1, 11) if i%2==0]
print(nums)
# output: [2, 4, 6, 8, 10]
Example 3:
Create a list of odd numbers from 1 to 10.
nums = [i for i in range(1, 11) if i%2!=0]
print(nums)
# output: [1, 3, 5, 7, 9]
Example 4:
Create a list by executing a given function on each element of a list.
def factorial(n):
if n == 0:
return 1
return n * factorial(n-1)
nums = [1, 2, 3, 4, 5]
factorialList = [factorial(i) for i in nums]
print(factorialList)
# output: [1, 2, 6, 24, 120]
Example 5:
Create a list of all strings with lengths between 2 and 5.
strList = ["HTML", "C++", "Python", "JavaScript", "Java"]
newList = [i for i in strList if len(i) in range(2, 6)]
print(newList)
# output: ['HTML', 'C++', 'Java']
Example 6:
Create a list of tuples from two lists.
num = [1, 2]
strList = ["C++", "Python"]
newList = [(i, j) for i in num for j in strList]
print(newList)
# output: [(1, 'C++'), (1, 'Python'), (2, 'C++'), (2, 'Python')]
Quiz - Python list comprehension tutorial quiz
[i for i in range(5)]
return?Conclusion
Using list comprehension can reduce the number of lines of code and also makes the code faster. It is a very useful tool to have in your Python arsenal.
Now that you have learned about list comprehension, you can use it in your code to make it more efficient.