Nested for loops in JavaScript
A Nested for loops are loops inside another loop. There can be any number of nested loops inside a loop.
The inner loop executes completely for each iteration of the outer loop.
For example, if the outer loop executes 5 times and the inner loop executes 2 times, then the inner loop will execute 5 × 2 = 10 times in total.
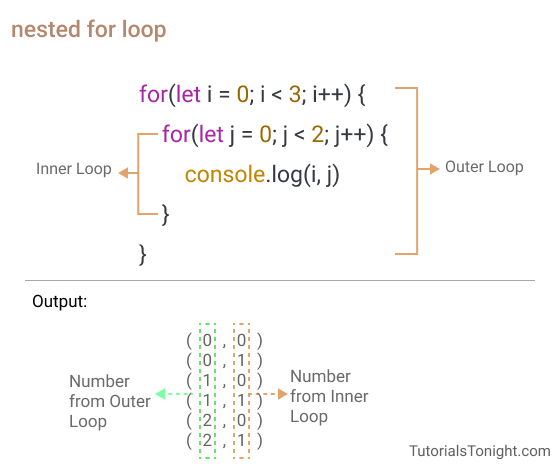
If there are 3 layers of nested loops, then the innermost loop will execute for the product of the number of iterations of the outer loops and middle loops.
For example, if the outer loop executes 5 times, the middle loop executes 3 times and the inner loop executes 2 times, then the innermost loop will execute 5 × 3 × 2 = 30 times in total.
Use of Nested Loops
In programming nested loops have many applications, some of which are listed below:
- Hanlding 2D arrays
- Generating all possible combinations of a set of values
- Pattern generation
- Search or compare elements of an array
- Sorting
- Matrix multiplication
- Tree traversal
- Image processing
- And many more...
Example: Nested for loop in JavaScript
Examples will teach you better than words. So, let's see some examples of nested for loops in JavaScript.
Example 1
A simple example of nested for loop to show the execution of the inner and outer loop.
Example
// Outer Loop
for (let i = 0; i < 3; i++) {
console.log(`Outer Loop: ${i}`);
// Inner Loop
for (let j = 0; j < 2; j++) {
console.log(` Inner Loop: ${j}`);
}
}
Output:
Outer Loop: 0 Inner Loop: 0 Inner Loop: 1 Outer Loop: 1 Inner Loop: 0 Inner Loop: 1 Outer Loop: 2 Inner Loop: 0 Inner Loop: 1
In the above output, you can see that the inner loop executes completely for each iteration of the outer loop.
Example 2
Let's see another example of nested for loop to print a table of numbers from 1 to 10.
Example
for (let i = 1; i <= 10; i++) {
console.log(`Table of ${i}`);
for (let j = 1; j <= 10; j++) {
console.log(`${i} × ${j} = ${i * j}`);
}
console.log();
}
Just click the run button above and a table of numbers from 1 to 10 will be printed in the console.
Example 3
In this example, we are creating a pyramid of stars using nested for loop.
Example
let size = 5;
let str = "";
// Outer loop
for (let i = 0; i < size; i++) {
// Inner loop to print spaces
for (let j = 0; j < size - i; j++) {
str += " ";
}
// Inner loop to print stars
for (let k = 0; k < i + 1; k++) {
str += "* ";
}
str += "\n";
}
console.log(str);
Output:
* * * * * * * * * * * * * * *
Example 4
Let's use nested for loops to create coordinates for a chessboard.
Example
let size = 8;
let str = "";
// Outer loop
for (let i = 0; i < size; i++) {
// Inner loop
for (let j = 0; j < size; j++) {
str += `(${i}, ${j}) `;
}
str += "\n";
}
console.log(str);
Output:
(0, 0) (0, 1) (0, 2) (0, 3) (0, 4) (0, 5) (0, 6) (0, 7) (1, 0) (1, 1) (1, 2) (1, 3) (1, 4) (1, 5) (1, 6) (1, 7) (2, 0) (2, 1) (2, 2) (2, 3) (2, 4) (2, 5) (2, 6) (2, 7) (3, 0) (3, 1) (3, 2) (3, 3) (3, 4) (3, 5) (3, 6) (3, 7) (4, 0) (4, 1) (4, 2) (4, 3) (4, 4) (4, 5) (4, 6) (4, 7) (5, 0) (5, 1) (5, 2) (5, 3) (5, 4) (5, 5) (5, 6) (5, 7) (6, 0) (6, 1) (6, 2) (6, 3) (6, 4) (6, 5) (6, 6) (6, 7) (7, 0) (7, 1) (7, 2) (7, 3) (7, 4) (7, 5) (7, 6) (7, 7)
Example 5
A great use of nested for loops is to generate all possible combinations of a set of values.
For example, if we have an array of 3 elements, then there are 33 = 27 possible combinations of these elements.
Let's see how we can generate all these combinations using nested for loops.
Example
let arr = ["A", "B", "C"];
let size = arr.length;
let str = "";
// Outer loop
for (let i = 0; i < size; i++) {
// Inner loop
for (let j = 0; j < size; j++) {
// Innermost loop
for (let k = 0; k < size; k++) {
str += `${arr[i]}${arr[j]}${arr[k]} `;
}
}
}
console.log(str);
console.log(`Total Combinations: ${size ** 3}`);
Output:
AAA AAB AAC ABA ABB ABC ACA ACB ACC BAA BAB BAC BBA BBB BBC BCA BCB BCC CAA CAB CAC CBA CBB CBC CCA CCB CCC
As you can see in the above output, there are 27 combinations of the elements of the array.
break and continue statements in Nested Loops
Just like a normal loop, we can use break and continue statements in nested loops.
These statements will help you control the flow of the nested loop to perform a specific task.
Let's see them one by one.
break statement in Nested Loops
The following example is using break statement to break out of the inner loop when the value of j is equal to 2.
When the value of j is equal to 2, the inner loop will break and the control will be transferred to the outer loop.
Then the outer loop will continue its execution and the inner loop will start again from the beginning.
When the value of j is equal to 2 again, the inner loop will break again and the control will be transferred to the outer loop.
This process will continue until the outer loop is finished.
Example
// Outer Loop
for (let i = 0; i < 2; i++) {
console.log(`Outer Loop: ${i}`);
// Inner Loop
for (let j = 0; j < 5; j++) {
if (j === 2) {
break;
}
console.log(` Inner Loop: ${j}`);
}
}
Output:
Outer Loop: 0 Inner Loop: 0 Inner Loop: 1 Outer Loop: 1 Inner Loop: 0 Inner Loop: 1
continue statement in Nested Loops
The following example uses the continue statement to skip the execution of the inner loop when the value of j is equal to 2.
In this case, when the value of j is equal to 2, the inner loop will skip the execution and jump to the next iteration.
Example
// Outer Loop
for (let i = 0; i < 2; i++) {
console.log(`Outer Loop: ${i}`);
// Inner Loop
for (let j = 0; j < 5; j++) {
if (j === 2) {
continue;
}
console.log(` Inner Loop: ${j}`);
}
}
Output:
Outer Loop: 0 Inner Loop: 0 Inner Loop: 1 Inner Loop: 3 Inner Loop: 4 Outer Loop: 1 Inner Loop: 0 Inner Loop: 1 Inner Loop: 3 Inner Loop: 4
Conclusion
You have now a good understanding of using nested for loop in JavaScript. Go through the examples, make some changes to them, write your own programs, adjust some values, and see the output.